Top 6 Useful Information That You Must Know About Django Template Tags 2021
What Are Django Template Tags?
Django Template Tagsare basic Python functions that accept one or more values, as well as an optional argument, and process them before returning a value to be shown on the page.
Create a "templatetags" directory at the same level as the models and views in your application folder.
Create an empty " init .py" file if you want this directory to be recognized as a Python package. Next, create a Python file called app tags.py that will house your tags.
The folder structure should be as shown in:
Django Project
my_app
models.py
views.py
templatetags
_init.py
app__tags__.py
Tags
Tags are formatted as follows: {% tag %}. Tags can be used to generate text in the output, control flow with loops and logic, and import external data into the template.
Beginning and ending tags are required for tags (i.e. {% tag %} ... tag contents ... {% endtag %}).
There are roughly twenty built-in template tags in Django. Here are a few of the most popular tags for - Each item in an array is looped over.
otherwise, elif, and if - Evaluates a variable and displays the contents of the block if the variable is "true."
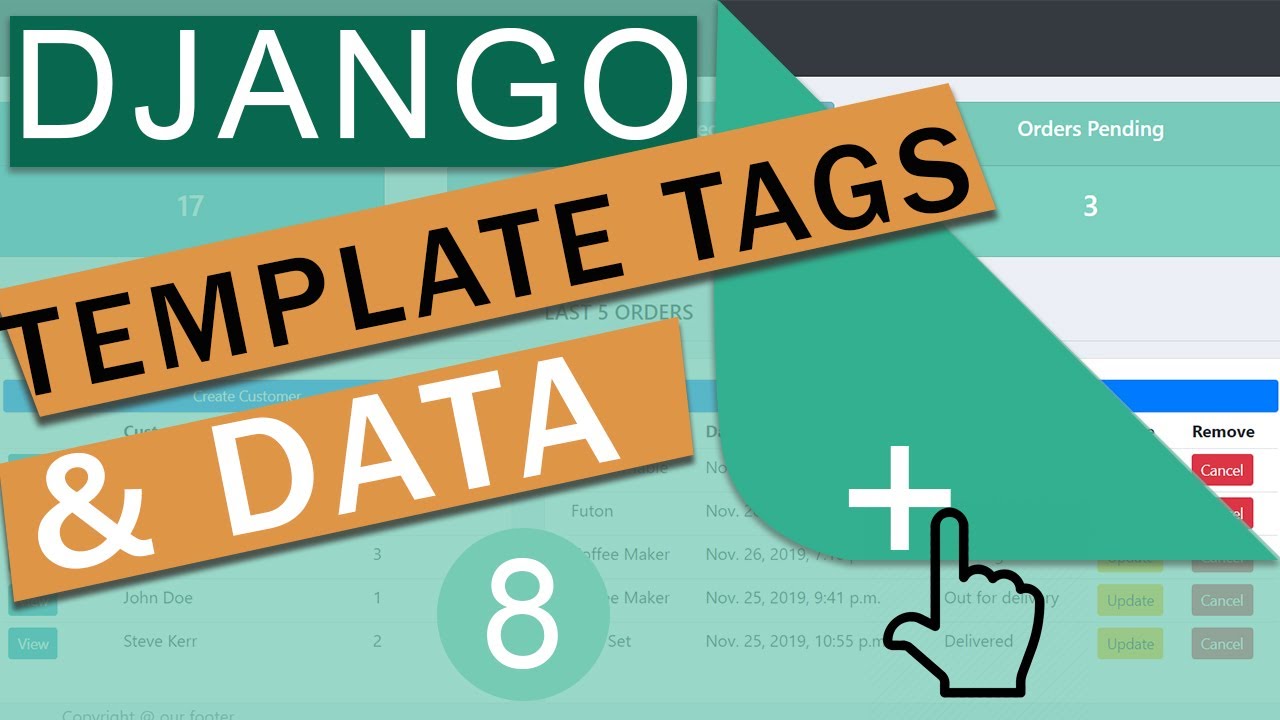
Rendering Data to Templates | Template Tags | Django (3.0) Crash Course Tutorials (pt 8)
Creating Custom Template Tags Of Your Own
From django import template:
register = template.Library(): This means that you must make sure that your templates are added to Django's current library of tags and filters. This statement creates a library instance from which you can register your filters. In this post, you'll learn how I register it with Django using the helpful "@register.assignment tag" decorator.
After enabling your custom tags, you may utilize the aforementioned template tag in your template by using "{% load app_tags %}".
An Example Of How To Incorporate These Functions Into Your Template Is As Follows
In your "example.html" template,
{% get_result_tag arg1 arg2 arg3 as result %}
The response from the template tag "get result tag" is provided by the aforementioned tag, which can be used with the variable "result."
Filters
We may alter the text, do mathematical operations, determine the file size, obtain the date, and so on using filters. Here are a few of the most common filters:
Length - To determine the length of a string or the size of a list. ({{ value|length }})
Date - Formats a date in the specified format. ( {{ value|date:"D d M Y" }}, {{ value|date:"m/d/Y H:i:s"}} )
File Size - To determine the file size in megabytes, use the Bytes command. ( {{ value|filesizeformat }} )
Sorting a Dictionary - Using a given key, sort a list of dictionaries. ( {{ value|dictsort:"country_id" }} )
The sample code for the custom filter may be found here.
This custom filter will be registered with Django using @register. I'm using this filter to retrieve the total number of modules in a chapter.
This filter can be used in templates like this:
{% chapter_id|get_modules:institute_id %}
You can also experiment with filters. I'm using numerous filters at the same time in this example.
Here, value1 and value2 are passed to the one more filter, which performs various operations before returning the data to the custom filter filter, which performs the operations.
How Do I Create A Custom Template Tag?
Django allows you to create a new tag to add functionality not covered by the built-in template tags. PyCharm offers you with the necessary code and navigation support.
Create the templatetags package in the application directory. (it should contain the init.py file). For example, Django/DjangoApp/templatetags.
Create a.py file, such as my custom tags, in the templatetags package, and add some code to it to declare a custom tag. More information about custom template tags coding practices may be found in the Django documentation.
To A Template, Add A Custom Template Tag
In the editor, open your template file and add the necessary template tag. Because you haven't loaded the custom tag into the template file, PyCharm displays the Unresolved tag inspection error.
To resolve the missing reference, use a quick-fix. Place your cursor above the custom tag name, click Alt+Enter, then choose the file you want to load.
To fix the reference, PyCharm adds the percent load percent tag to the template file.
PyCharm also recognizes custom template tags that are saved as built in tags in the settings.py file's templates section.
You can navigate to the file with the declaration of a custom tag once you've recorded it in the settings file. Ctrl+B while the cursor is over the template tag filename.
How Do I Add A Template To Django Project?
Go to the settings.py file and change the DIRS to the path of the templates folder to configure the Django template system. In most cases, the templates folder is created and retained in the sample directory, which also contains manage.py. This folder contains all of the templates you'll need in your various Django Apps.
Alternatively, you can keep a distinct template folder for each program. You don't need to adjust the DIRS in settings.py if you're maintaining the template for each app separately. Make sure your app is listed in settings.py's INSTALLED_APPS.
What Does The Built In Django Template Tag URL Do?
Django templates are text documents or Python strings that have been marked up with the Django template language. Django, as a powerful Batteries-included framework, makes rendering data in a template simple. Django templates not only allow data to be passed from view to template, but they also provide some programming elements such as variables, for loops, comments, extends, and urls.
The purpose of this article is to explain how to use the url tag in templates. Returns an absolute path reference (a URL without the domain name) that matches a view and optional parameters. This is a way to produce links without having to hard-code URLs in your templates, which violates the DRY principle:
{% url 'some-url-name' v1 v2 %}
The first argument is the name of a URL pattern. It could be a quoted literal or a context variable of any kind. Optional additional arguments should be space-separated values that will be utilized as arguments in the URL.
How Do Templates Work In Django?
Django's template system makes it simple to create dynamic HTML pages.
A template is made up of both static sections of the desired HTML output and specific syntax that describes how dynamic content will be included.
We can't put python code in an HTML file since the code is only interpreted by the Python interpreter, not the browser. HTML is a static markup language, but Python is a dynamic programming language, as we all know.
The Django template engine allows us to develop dynamic web pages by separating the design from the python code.
Django Template Variable
Template
A text file is what a template is. It is capable of producing any text-based format (HTML, XML, CSV, etc.).
Variables are used to substitute values when the template is evaluated, and tags are used to govern the logic of the template.
A simple template that demonstrates a few fundamentals is provided below. Each part will be discussed in further detail later in this document.
Below is the continuation of the template indicated above:
Variables
Variables are written like this: {{ variable }}.. When a variable is encountered, the template engine evaluates it and replaces it with the result. Variable names can contain any combination of alphanumeric letters and the underscore ("_"), but they must not begin with an underscore or be a number. In variable parts, the dot (".") also appears, but it has a unique meaning, as shown below. Importantly, variable names cannot contain spaces or punctuation characters.
To access a variable's attributes, type a dot (.).
The title attribute of the section object will be substituted for {{ section.title }} in the following example.
If you use a variable that doesn't exist, the template system will use the default value for the string if invalid option, which is " (the empty string).
It's worth noting that in a template expression like {{ foo.bar }}, "bar" is treated as a literal string rather than the value of the variable "bar," if one exists in the template context.
Variable properties that begin with an underscore are normally considered private and cannot be accessed.
Filters can be used to change the display of variables.
{{ name|lower }} is an example of a filter. This shows the value of the name variable after it has been filtered by the lower filter, which lowercases the text. To apply a filter, use a pipe (|).
Filters can be "chained" together. One filter's output is applied to the next. The idiom {{ text|escape|linebreaks }} is used to escape text contents before converting line breaks to < p > tags.
Some filters take arguments into account. The following is an example of a filter argument: {{ bio|truncatewords:30 }}. The first 30 words of the bio variable will be displayed.
Filter arguments containing spaces must be quoted; for example, to join a list of commas and spaces, use {{ list|join:", " }}.
There are roughly sixty built-in template filters in Django. The built-in filter reference will tell you everything you need to know about them.
Tags
Tags are formatted as follows: {% tag %} Tags are more difficult to understand than variables: Some generate text in the output, while others manage flow with loops or logic, and still others load external data into the template for later variables to use.
Some tags (i.e. {% tag %} ... tag contents ... {% endtag %}) require starting and ending tags.
Django comes with around a half-dozen template tags pre-installed. The built-in tag reference will tell you everything you need to know about them.